SEBA board class 10 computer science chapter 4 Introduction to Loops exercise and additional questions answers
SEBA board class 10 computer science chapter 4 introduction to Loops exercise and additional questions answers are given below in a simple language –
Exercise Questions:
Q. 1. Why do we use a loop in a C program ?
Ans: We use a loop in a C program to avoid typing the same set of statements again and again. Moreover using loop in a C program reduces the length of a program.
Q.2. Do we need to use only one type of loop in a C program ? justify your answer by writing a C program.
Ans: No. It is not mandatory to use only one type of loop in a C program . We can use all the types of loop in a program.
The above can be justified with the following program-
#include<stdio.h>
int main()
{
int i=1,j=1,n;
do{
printf(“Elearners mentor is an elearning platform for students\n”);
i=i+1;
}while(i<=2);
while(j<=2)
{
printf(“elearners mentor is an elearning platform for students\n”);
j=j+1;
}
for(n=1;n<=2;n++)
{
printf(“elearners mentor is an elearning platform for students\n”);
}
return 0;
}
Q.3. What will happen if we write a while loop with 1 in place of the condition ? Try it in a simple C program.
Hint :
while (1)
{
printf(“We must raise our voice against corruption \n”);
}
Ans: If we write a while loop with 1 in place of the condition, the loop will run infinite times because 1 in the condition expression means the condition is always true so the program will never terminate until we manually stop it.
4. Name different portions of a for loop. Can we put more than one statement within a portion ?
Ans: The different portions of a for loop are
i. Initialization expression
ii. Condition checking or testing expression
iii. Update expression.
Yes, we can put more than one statement within a portion.
5. Answer with True or False.
i. If the condition of the while loop is false, the control comes to the second statement inside the loop.
Ans: False.
ii. We can use atmost three loops in a single C program.
Ans: False.
iii. The statements inside the do-while loop executes atleast once even if the condition is false.
Ans: True.
iv. Only the first statement inside the do-while loop executes when the condition is false.
Ans: False.
v. In a do-while loop, the condition is written at the end of the loop.
Ans: False.
6. Programming exercise:
A. Write a C program to find the summation of the following series-
(a) 12+ 22 + 32+ 42+…………+N2
Ans:
#include<stdio.h>
int main()
{
int i, n, sum=0;
printf(“Enter how many numbers to be added”);
scanf(“%d”,&n);
for(i=1;i<=n;i++)
{
sum=sum+i*i;
}
printf(“The summation of the series is =%d”,sum);
return 0;
}
(b) 13 + 23 + 33 + 43 + ……………+ N3
Ans:
#include<stdio.h>
int main()
{
int i, n, sum=0;
printf(“Enter how many numbers to be added”);
scanf(“%d”,&n);
for(i=1;i<=n;i++)
{
sum=sum+i*i*i ;
}
printf(“The summation of the series is =%d”,sum);
return 0;
}
(c) 1*2 + 2*3 + 3*4 + ……….. + N*(N+1)
Ans:
#include<stdio.h>
int main()
{
int n,i,sum=0;
for(i=1;i<=5;i++)
{
sum=sum + i*(i+1);
}
printf(“The summation of the series is =%d”,sum);
return 0;
}
B. Write a C program to continuously take a number as input and announce whether the number is odd or even. Hint : use do – while loop.
Ans:
#include<stdio.h>
int main()
{
int i=1,n;
do
{
printf(“Enter a number: “);
scanf(“%d”,&n);
if(n%2==0)
{
printf(“%d is an even number\n”,n);
}
else
{
printf(“%d is an odd number\n”,n);
}
i=i+1;
}while(i<=5);
return 0;
}
C. Write a program to display the following pattern.
1
1 1
1 1 1
1 1 1 1
1 1 1 1 1
Ans: #include<stdio.h>
int main()
{
int i;
for(i=1;i<=1;i++)
{
printf(“1”);
}
printf(“\n”);
for(i=1;i<=2;i++)
{
printf(“1”);
}
printf(“\n”);
for(i=1;i<=3;i++)
{
printf(“1”);
}
printf(“\n”);
for(i=1;i<=4;i++)
{
printf(“1”);
}
printf(“\n”);
for(i=1;i<=5;i++)
{
printf(“1”);
}
printf(“\n”);
return 0;
}
D. Write a C program to display the following pattern.
5
5 4
5 4 3
5 4 3 2
5 4 3 2 1
Ans: #include<stdio.h>
int main()
{
int i;
for(i=5;i>=5;i–)
{
printf(“%d”,i);
}
printf(“\n”);
for(i=5;i>=4;i–)
{
printf(“%d”,i);
}
printf(“\n”);
for(i=5;i>=3;i–)
{
printf(“%d”,i);
}
printf(“\n”);
for(i=5;i>=2;i–)
{
printf(“%d”,i);
}
printf(“\n”);
for(i=5;i>=1;i–)
{
printf(“%d”,i);
}
printf(“\n”);
return 0;
}
E. Write a C program to display the following pattern.
5 4 3 2 1
5 4 3 2
5 4 3
5 4
5
Ans: #include<stdio.h>
int main()
{
int i;
for(i=5;i>=1;i–)
{
printf(“%d”,i);
}
printf(“\n”);
for(i=5;i>=2;i–)
{
printf(“%d”,i);
}
printf(“\n”);
for(i=5;i>=3;i–)
{
printf(“%d”,i);
}
printf(“\n”);
for(i=5;i>=4;i–)
{
printf(“%d”,i);
}
printf(“\n”);
for(i=5;i>=5;i–)
{
printf(“%d”,i);
}
printf(“\n”);
return 0;
}
*****
Dear students if you want the complete explanation on this chapter, visit my YouTube channel
Related Posts
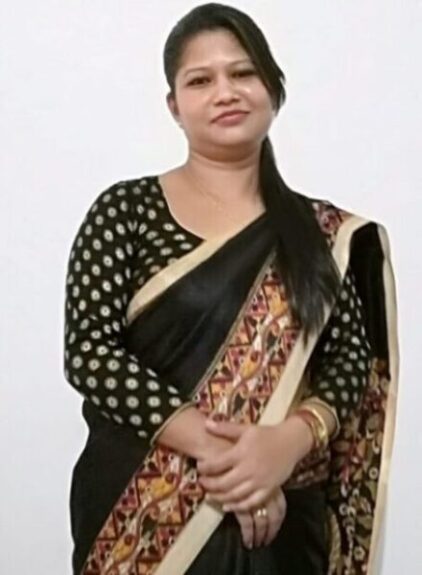
Hello students,
Welcome to my site. I am the founder of the blog elearnersmentor.com. I have been in teaching profession since 2004. Presently I am working as a vocational subject teacher(IT) in a reputed govt. higher secondary school. Read more